ell::LT_MinimaSet Class Reference
#include <LT_MinimaSet.hh>
Inheritance diagram for ell::LT_MinimaSet:
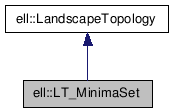
Detailed Description
Definition at line 14 of file LT_MinimaSet.hh.
Public Member Functions | |
virtual const size_t | addMin (const State *const m) |
virtual bool | addSaddle (const State *const m_i, const State *const m_j, const State *const s) |
virtual bool | addSaddle (const size_t i, const size_t j, const State *const s) |
virtual void | clear () |
virtual const std::vector< const State * > & | getAllMin () const |
virtual const State *const | getMFEState () const |
virtual const State *const | getMin (size_t i) const |
virtual const size_t | getMinCount () const |
virtual const size_t | getMinIndex (const State *const m) const |
virtual double | getRMSD (const LandscapeTopology *const lt) const |
virtual bool | isSorted () const |
LT_MinimaSet () | |
virtual std::pair< int, std::string > | read (std::istream &in, const State *const templateState, const std::string &StateDescription) |
virtual void | sort () |
virtual void | write (std::ostream &out, const std::string &StateDescription) const |
virtual | ~LT_MinimaSet () |
Static Public Attributes | |
static const size_t | INVALID_INDEX |
static const std::string | LT_ID |
Static Protected Member Functions | |
static bool | isSmaller_E_S (const State *a, const State *b) |
Protected Attributes | |
size_t | mfeIndex |
bool | sorted |
std::vector< const State * > | vMinima |
Constructor & Destructor Documentation
ell::LT_MinimaSet::LT_MinimaSet | ( | ) |
ell::LT_MinimaSet::~LT_MinimaSet | ( | ) | [virtual] |
Member Function Documentation
const size_t ell::LT_MinimaSet::addMin | ( | const State *const | m | ) | [virtual] |
Adds an UNKNOWN minimum to the topology. NOTE : this operation should NOT change the indices of already existing minima in the topology ! FURTHER : the minimum is not checked if it is already known
- Parameters:
-
m the first minimum to add
- Returns:
- the index of the minimum in the landscape
Implements ell::LandscapeTopology.
Definition at line 46 of file LT_MinimaSet.cc.
bool ell::LT_MinimaSet::addSaddle | ( | const State *const | m_i, | |
const State *const | m_j, | |||
const State *const | s | |||
) | [virtual] |
Sets a saddle point that connects two minima
- Parameters:
-
m_i the first minimum to connect m_j the second minimum to connect s the State representating the saddle point
- Returns:
- whether or not the data structure has been changed
Implements ell::LandscapeTopology.
Definition at line 40 of file LT_MinimaSet.cc.
bool ell::LT_MinimaSet::addSaddle | ( | const size_t | i, | |
const size_t | j, | |||
const State *const | s | |||
) | [virtual] |
Sets a saddle point that connects two minima
- Parameters:
-
i index of the first minimum to connect j index of the second minimum to connect s the State representating the saddle point
- Returns:
- whether or not the data structure has been changed
Implements ell::LandscapeTopology.
Definition at line 34 of file LT_MinimaSet.cc.
void ell::LT_MinimaSet::clear | ( | ) | [virtual] |
Clears the data structure and removes all stored information to be filled again afterwards.
Implements ell::LandscapeTopology.
Definition at line 21 of file LT_MinimaSet.cc.
const std::vector< const State * > & ell::LT_MinimaSet::getAllMin | ( | ) | const [virtual] |
Access to all minima.
- Returns:
- a vector representation of all minima
Definition at line 105 of file LT_MinimaSet.cc.
const State *const ell::LT_MinimaSet::getMFEState | ( | ) | const [virtual] |
Access to the global minimum of the landscape with minimal free energy (mfe).
- Returns:
- the minimum with smallest energy (and smallest string representation for tiebreaking)
Implements ell::LandscapeTopology.
Definition at line 99 of file LT_MinimaSet.cc.
const State *const ell::LT_MinimaSet::getMin | ( | size_t | i | ) | const [virtual] |
Access to a minimum.
- Parameters:
-
i index of the minimum to access
- Returns:
- the State with the specified minimum index
Implements ell::LandscapeTopology.
Definition at line 74 of file LT_MinimaSet.cc.
const size_t ell::LT_MinimaSet::getMinCount | ( | ) | const [virtual] |
Access to the number of stored minima.
- Returns:
- the number of minima
Implements ell::LandscapeTopology.
Definition at line 93 of file LT_MinimaSet.cc.
const size_t ell::LT_MinimaSet::getMinIndex | ( | const State *const | m | ) | const [virtual] |
Access to the minimum index.
- Parameters:
-
m the minimum of that the index should be accessed
- Returns:
- the index of the minimum, or INVALID_INDEX if 'm' is no known minimum
Implements ell::LandscapeTopology.
Definition at line 82 of file LT_MinimaSet.cc.
double ell::LT_MinimaSet::getRMSD | ( | const LandscapeTopology *const | lt | ) | const [virtual] |
Calculates the root mean square deviation between landscape topologies.
- Parameters:
-
lt the landscape topology to calculate the RMSD
- Returns:
- the RMSD
Implements ell::LandscapeTopology.
Definition at line 112 of file LT_MinimaSet.cc.
bool ell::LandscapeTopology::isSmaller_E_S | ( | const State * | a, | |
const State * | b | |||
) | [static, protected, inherited] |
Comparison function that compares on energy and string representation
- Returns:
- true if energy of a smaller than b or if equal if the string represenation of a is lexicographically smaller than b
Definition at line 10 of file LandscapeTopology.cc.
bool ell::LT_MinimaSet::isSorted | ( | ) | const [virtual] |
Check if the minima of the landscape are sorted ascendingly by their energy (mfe minimum gets index 0). If energy is equal they are sorted by their string representation.
Implements ell::LandscapeTopology.
Definition at line 157 of file LT_MinimaSet.cc.
std::pair< int, std::string > ell::LT_MinimaSet::read | ( | std::istream & | in, | |
const State *const | templateState, | |||
const std::string & | StateDescription | |||
) | [virtual] |
Fills the landscape topology from stream.
- Parameters:
-
in the input stream to read from. templateState the State template to create new State objects via its 'fromString' function StateDescription a string that describes the type of states that should be read and that is compared to the LT information header in the stream
- Returns:
- an error encoding and an error string : 0 = no error occured, read was successfull -1 = landscape topology type not supported -2 = state description differs from the given one -3 = other read errors
first step: get the type information for the saddle net( invisible for graphviz as comments //)
second step: get all the minima and saddles (graphviz comments with // )
if graphViz data starts.. end the reading
Implements ell::LandscapeTopology.
Definition at line 162 of file LT_MinimaSet.cc.
void ell::LT_MinimaSet::sort | ( | ) | [virtual] |
Sorts the minima ascending by their energy (mfe minimum gets index 0). If energy is equal they are sorted by their string representation.
Implements ell::LandscapeTopology.
Definition at line 144 of file LT_MinimaSet.cc.
void ell::LT_MinimaSet::write | ( | std::ostream & | out, | |
const std::string & | StateDescription | |||
) | const [virtual] |
Writes the current landscape topology to stream.
- Parameters:
-
out the output stream to write to. StateDescription a string that describes the type of states written to the stream (will be part of the LT information header)
step 1: save the datastructure for later reconstruction as comments in graphviz file
step2: put all minima in with.. MINIMUM= index toString()-format
step 2: write the graph in DOT language
Implements ell::LandscapeTopology.
Definition at line 274 of file LT_MinimaSet.cc.
Field Documentation
const size_t ell::LandscapeTopology::INVALID_INDEX [static, inherited] |
Index value that is used to propagate the information about invalid indexing.
Definition at line 32 of file LandscapeTopology.hh.
const std::string ell::LT_MinimaSet::LT_ID [static] |
the landscape topology id string used in the output header to identify a minima set
Definition at line 28 of file LT_MinimaSet.hh.
size_t ell::LT_MinimaSet::mfeIndex [protected] |
index of the global minimum (with minimal free energy)
Definition at line 20 of file LT_MinimaSet.hh.
bool ell::LT_MinimaSet::sorted [protected] |
internal flag that stores if vMinima is sorted or not
Definition at line 22 of file LT_MinimaSet.hh.
std::vector<const State*> ell::LT_MinimaSet::vMinima [protected] |
The documentation for this class was generated from the following files:
- src/ell/LT_MinimaSet.hh
- src/ell/LT_MinimaSet.cc