biu::RNAStructure Class Reference
#include <RNAStructure.hh>
Inheritance diagram for biu::RNAStructure:
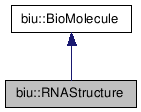
Detailed Description
An object of the class RNAStructure represents a RNA as a BioMolecule.An object of this class is allowed to have only nested bonds.
Definition at line 18 of file RNAStructure.hh.
Public Member Functions | |
size_t | getClosingBond (size_t openingBond) const |
virtual double | getEnergy () const=0 |
size_t | getLength () const |
size_t | getMinLoopLength () const |
Sequence | getSequence () const |
std::string | getSequenceString () const |
virtual std::string | getStringRepresentation () const |
Structure | getStructure () const |
const Structure & | getStructureRef () const |
std::string | getStructureString () const |
bool | hasMinLoopLength () const |
bool | hasValidBasePairs () const |
bool | hasValidStructure () const |
bool | isAllowedBasePair (size_t first, size_t second) const |
virtual bool | isValid () const |
bool | operator!= (const RNAStructure &rnaStruct2) const |
RNAStructure & | operator= (const RNAStructure &rnaStruct2) |
bool | operator== (const RNAStructure &rnaStruct2) const |
RNAStructure (const RNAStructure &rnaStruct) | |
RNAStructure (Sequence *rnaSeq, const Structure *const rnaStructBracketDot, const AllowedBasePairs *const bPair, const bool seqIsShared) | |
RNAStructure (const std::string &rnaSeqStr, const std::string &rnaStructBracketDotStr, const AllowedBasePairs *const bPair) | |
virtual void | setStructure (const Structure &str) |
virtual | ~RNAStructure () |
Static Public Member Functions | |
static const Alphabet * | getStructureAlphabet () |
Static Public Attributes | |
static const size_t | INVALID_INDEX |
static const Alphabet::AlphElem | STRUCT_BND_CLOSE |
static const Alphabet::AlphElem | STRUCT_BND_OPEN |
static const Alphabet::AlphElem | STRUCT_UNBOUND |
Protected Member Functions | |
void | initBonds () |
Protected Attributes | |
const AllowedBasePairs * | bPair |
std::vector< size_t > | rnaBonds |
Sequence * | rnaSeq |
Structure * | rnaStructBracketDot |
const bool | seqShared |
Static Protected Attributes | |
static const size_t | MIN_LOOP_LENGTH |
static const Alphabet | STRUCT_ALPH |
Constructor & Destructor Documentation
biu::RNAStructure::RNAStructure | ( | const std::string & | rnaSeqStr, | |
const std::string & | rnaStructBracketDotStr, | |||
const AllowedBasePairs *const | bPair | |||
) |
Construction of an RNA molecule
It is explicitly allowed to construct invalid RNAStructures, but each bond has to be complete (each opening has a closing bracket).
Using this constructor no sequence sharing is possible.
- Parameters:
-
rnaSeqStr is the RNA sequence rnaStructBracketDotStr is the RNA structure in bracket notation. bPair is the handler for the allowed base pairs.
Definition at line 20 of file RNAStructure.cc.
biu::RNAStructure::RNAStructure | ( | Sequence * | rnaSeq, | |
const Structure *const | rnaStructBracketDot, | |||
const AllowedBasePairs *const | bPair, | |||
const bool | seqIsShared | |||
) |
Construction of an RNA molecule
It is explicitly allowed to construct invalid RNAStructures, but each bond has to be complete (each opening has a closing bracket).
- Parameters:
-
rnaSeq the RNA sequence representation rnaStructBracketDot the RNA structure representation in bracket notation. bPair is the handler for the allowed base pairs. seqIsShared whether or not the rnaSeqStr representation should be shared among all derived objects of this class and no copy should be done
Definition at line 47 of file RNAStructure.cc.
biu::RNAStructure::RNAStructure | ( | const RNAStructure & | rnaStruct | ) |
Definition at line 80 of file RNAStructure.cc.
biu::RNAStructure::~RNAStructure | ( | ) | [virtual] |
Definition at line 95 of file RNAStructure.cc.
Member Function Documentation
size_t biu::RNAStructure::getClosingBond | ( | size_t | openingBond | ) | const [inline] |
Returns the corresponding closing bond index to the given opening index
Returns INVALID_INDEX in error case, if there doesnt exist a closing bond.
Definition at line 132 of file RNAStructure.hh.
virtual double biu::BioMolecule::getEnergy | ( | ) | const [pure virtual, inherited] |
Returns the specific energy of the BioMolecule.
Implemented in biu::LatticeProtein_Ipnt, and biu::RNAStructure_TB.
size_t biu::RNAStructure::getLength | ( | ) | const [inline, virtual] |
Returns the length of the Biomolecule, i.e. the number of monomers.
Implements biu::BioMolecule.
Definition at line 161 of file RNAStructure.hh.
size_t biu::RNAStructure::getMinLoopLength | ( | ) | const [inline] |
Definition at line 136 of file RNAStructure.hh.
Sequence biu::RNAStructure::getSequence | ( | ) | const [inline, virtual] |
std::string biu::RNAStructure::getSequenceString | ( | ) | const [inline] |
Definition at line 140 of file RNAStructure.hh.
std::string biu::RNAStructure::getStringRepresentation | ( | ) | const [virtual] |
Returns a combination of bracket notation and sequence information of this RNA.
BRACKETNOTATION(SEQUENCE)
Implements biu::BioMolecule.
Definition at line 211 of file RNAStructure.cc.
Structure biu::RNAStructure::getStructure | ( | ) | const [inline, virtual] |
Returns the RNA structure in bracket notation.
Implements biu::BioMolecule.
Definition at line 167 of file RNAStructure.hh.
static const Alphabet* biu::RNAStructure::getStructureAlphabet | ( | ) | [inline, static] |
Definition at line 148 of file RNAStructure.hh.
const Structure& biu::RNAStructure::getStructureRef | ( | ) | const [inline] |
Definition at line 181 of file RNAStructure.hh.
std::string biu::RNAStructure::getStructureString | ( | ) | const [inline] |
Definition at line 144 of file RNAStructure.hh.
bool biu::RNAStructure::hasMinLoopLength | ( | ) | const |
Returns whether all loops of an RNAStructure object have at least a length of MIN_LOOP_LENGTH.
Definition at line 190 of file RNAStructure.cc.
bool biu::RNAStructure::hasValidBasePairs | ( | ) | const |
Returns whether all RNA base pairs of an RNAStructure object are valid.
Definition at line 178 of file RNAStructure.cc.
bool biu::RNAStructure::hasValidStructure | ( | ) | const |
Returns whether the RNA structure is in valid bracket notation (each opening has a closing bracket).
Definition at line 160 of file RNAStructure.cc.
void biu::RNAStructure::initBonds | ( | ) | [protected] |
Initialises the bonds data structure. (called from constructors).
Definition at line 216 of file RNAStructure.cc.
bool biu::RNAStructure::isAllowedBasePair | ( | size_t | first, | |
size_t | second | |||
) | const |
Returns whether a base pair between positions first and second is valid.
Definition at line 105 of file RNAStructure.cc.
bool biu::RNAStructure::isValid | ( | ) | const [virtual] |
Returns true if an RNAStructure object has a valid structure with valid base pairs and loops of a length of at least MIN_LOOP_LENGTH.
Implements biu::BioMolecule.
Definition at line 203 of file RNAStructure.cc.
bool biu::RNAStructure::operator!= | ( | const RNAStructure & | rnaStruct2 | ) | const |
Definition at line 151 of file RNAStructure.cc.
RNAStructure & biu::RNAStructure::operator= | ( | const RNAStructure & | rnaStruct2 | ) |
Definition at line 116 of file RNAStructure.cc.
bool biu::RNAStructure::operator== | ( | const RNAStructure & | rnaStruct2 | ) | const |
Definition at line 142 of file RNAStructure.cc.
virtual void biu::RNAStructure::setStructure | ( | const Structure & | str | ) | [inline, virtual] |
Sets the RNA structure in bracket notation.
- Parameters:
-
str the structure to set in bracket notation of correct length
Definition at line 175 of file RNAStructure.hh.
Field Documentation
const AllowedBasePairs* biu::RNAStructure::bPair [protected] |
A pointer to an BasePair object containing the valid RNA base pairs
Definition at line 49 of file RNAStructure.hh.
const size_t biu::RNAStructure::INVALID_INDEX [static] |
Constant that represents a invalid structure index position.
Definition at line 61 of file RNAStructure.hh.
const size_t biu::RNAStructure::MIN_LOOP_LENGTH [static, protected] |
std::vector<size_t> biu::RNAStructure::rnaBonds [protected] |
Mapping from index of an opening bond to the index of the corresponding closing bond Entry at index i is index of the closing bond and INVALID_INDEX if index i is not an opening bond
Definition at line 44 of file RNAStructure.hh.
Sequence* biu::RNAStructure::rnaSeq [protected] |
Structure* biu::RNAStructure::rnaStructBracketDot [protected] |
const bool biu::RNAStructure::seqShared [protected] |
const Alphabet biu::RNAStructure::STRUCT_ALPH [static, protected] |
The alphabet of the structure representation which contains the left and right parenthesis and a dot.
Definition at line 34 of file RNAStructure.hh.
const Alphabet::AlphElem biu::RNAStructure::STRUCT_BND_CLOSE [static] |
const Alphabet::AlphElem biu::RNAStructure::STRUCT_BND_OPEN [static] |
const Alphabet::AlphElem biu::RNAStructure::STRUCT_UNBOUND [static] |
The documentation for this class was generated from the following files:
- src/biu/RNAStructure.hh
- src/biu/RNAStructure.cc